Automated Evocations
For the module to work correctly, players need world level permission to create tokens.
A user interface to manage companions with summoning animations and automated summoning for spells. The Companion Manager works on all Systems, while the automations only work on DnD5e, PF2E. To configure automations on other systems check Manually invoking the companion manger on spell cast. The module includes a Macro and Actor compendium with preconfigured summons.
How to use

Companion Manager
Open any character sheet, in the header of the window you will see the companions button Upon opening you will be welcomed by a window, from here you can drag and drop actors from the sidebar or compendiums into it to add them. After adding actor to the window you will have some options:
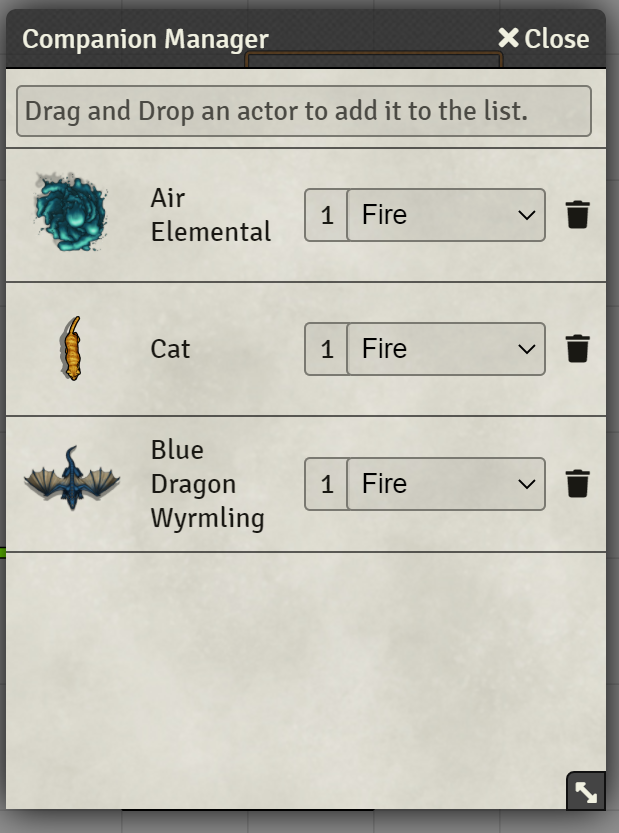
- To Summon : click on the actor image, you will get a placement croshair, just click where you want to summon the token
- Number field: how many tokens you will spawn
- Animation: The dropdown will let you chose the summoning animation
Store companions on actor
By default companions are stored per user (so each actor will have the same summon list). You can set per actor storage in the module settings if you want each different actor to have it's own companion list.
Advanced
If you want a particular actor to have it's own summon list you can use
the included macro to switch the actor from global storage to local (on the actor).
Simply place a linked actor on the scene, select it and run the macro.
Using the other macro to switch it to global again will not wipe the saved companions so setting it to local at a later date will restore the previous list.
For more advanced users you can set the flag with the following command : actor.setFlag(AECONSTS.MN,"isLocal",false)
(set true/false to enable disable local storage)
Custom Macros
You can assign custom macros to specific actors.
The steps are as follows:
- Create a macro with this naming structure
AE_Companion_Macro(ActorName)
whereActorName
is replaced with the actor's name.- A macro for an actor named Bat would be
AE_Companion_Macro(Bat)
- This will get fired any time a creature with that name is summoned
- A macro for an actor named Bat would be
- Add code for the custom data, in the context of the macro
args[0]
contains the following data:
Data | Description | Notes |
---|---|---|
summon | The actor that's getting summoned | |
spellLevel | The level of the spell that triggered the summoning | |
duplicates | How many creatures are getting summoned | |
assignedActor | The actor assigned to the player doing the summoning | This will be the selected token actor if no assigned actor is found, this is always the case for GMs. The macro must return the custom data. |
You can use the getSummonInfo
API call (CompanionManager.api.getSummonInfo
), passing in args
and the base spell level, to get the following information automatically calculated for you:
Data | Description |
---|---|
level | How many levels above the base spell level the spell as cast at |
maxHP | The actor's max HP |
dc | The actor's spellcasting DC |
attack.ms | The melee spell attack bonus of the actor |
attack.rs | The ranged spell attack bonus of the actor |
An example using Flaming Sphere with auto scaling:
// Macro name: AE_Companion_Macro(Flaming Sphere)
const summon = CompanionManager.api.dnd5e.getSummonInfo(args, 2);
const flamingSphere = {
sphere: [`${summon.level + 2}d6`, "fire"],
};
return {
embedded: {
Item: {
"Flaming Sphere": {
"data.description.value": `Any creature that ends its turn within 5 feet of the sphere, or has the sphere rammed into it, must make a Dexterity saving throw (DC ${summon.dc}). The creature takes ${flamingSphere.sphere[0]} ${flamingSphere.sphere[1]} damage on a failed save, or half as much damage on a successful one.`,
"data.save.dc": summon.dc,
"data.damage.parts": [flamingSphere.sphere],
},
},
},
};
Every time an
actor named Arcane Hand
is summoned, the custom data will be applied
Supported Spells
To use the included automations you will need to import both the Actor and the corresponding Macro from Automated Evocation compendiums! The ever expanding list of spells currently includes: All the SRD spells for dnd5e, if something is missing let me know
Custom and non-SRD spells
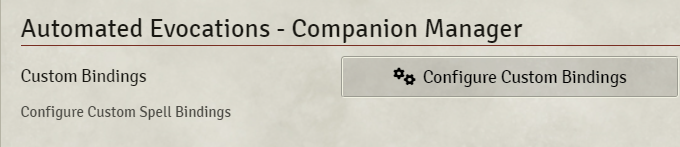
To add your custom spell bindings use the Custom Bindings setting in the module settings.
- Open the
Configure Custom Bindings
menuCustom Bindings Menu - Click
Add Binding
- Rename the binding with the name of the spell or feature you want to bind by clicking on the name. This is
Case Sensitive
- Click the
Edit
button, a new window will open where you can configure this Binding - Click the
Add Binding
button to add a new creature - Edit the creature name to match the creature. This is
Case Sensitive
- Edit the number to summon and the animation.
- Close the windows.
Custom Animations
To add your own animations, you can merge your own
configs to the default one. Once you built the object you wanna merge,
simply save it to the hidden game setting
game.settings.set(AECONSTS.MN, "customanimations", yourData)
Setting this hidden setting will override any previous value, so you want to keep a file with all you custom setting and add to it every time you want to apply it!
Example: Adding your animation to the list:
const customanims = {
energy2: {
fn: "light2",
time: 650,
name: "Energy 2",
group: "My Group", //optional
},
};
game.settings.set(AECONSTS.MN, "customanimations", customanims);
fn
: name of the macro to firetime
: how long to wait from the animation start before spwaning the tokenname
: the displayed name
Example macro:
const template = args[0];
const tokenData = args[1];
const tokenScale = (Math.abs(tokenData.texture.scaleX) + Math.abs(tokenData.texture.scaleY)) / 2;
await new Sequence()
.effect()
.file("modules/automated-evocations/assets/animations/energy\_spark\_CIRCLE\_01.webm")
.belowTokens()
.randomRotation()
.atLocation(template, { randomOffset: true })
.repeats(6,50, 25, 75, 60,20)
.scale(Math.max(tokenData.width,tokenData.height) * tokenScale * 0.15)
.wait(500)
.effect()
.file("modules/automated-evocations/assets/animations/energy\_pulse\_yellow\_CIRCLE.webm")
.belowTokens().atLocation(template)
.scale(Math.max(tokenData.width,tokenData.height) * tokenScale * 0.35)
.play()
Manually invoking the companion manger on spell cast
If you are on non DND5E systems you can trigger the companion manager for specific spells with a macro or the module Item Macro
new SimpleCompanionManager(
[
{
id: "actorid", //id of the actor to summon, if you have the name use game.actors.getName(name).id
animation: "animationid", //id of the animation - set to undefined for
defaultnumber: 1, //number of creatures to spawn
},
{
id: "actorid",
animation: "animationid",
number: 1,
},
],
spellLevel,
actor
).render(true);
//spell level is the spell level of the spell that summons the companions (will be passed to the companion macro), actor is the actor that summons the companions
Credits
- PF2E Support : TomChristoffer#6777
- Jack Kerouac's: The Fire, Air, Lightning, Water, Energy, Magic, Heart, Crescendo, Four Elements animations assets are from Jack Kerouac's amazing Animated Spell Effects: Cartoon (opens in a new tab) module with his permission.
- JB2A: The Chord, Darkness, Ice, Conjuration, and Storm animations assets are courtesy of JB2A with their permission. Check out their patreon (opens in a new tab) and discord (opens in a new tab) for many more amazing animations and variations.
- Sequencer (opens in a new tab): This module is used to play the animations
- Warpgate (opens in a new tab) Warpgate is used for spawning.
- Game Icons (opens in a new tab) Some images used are from Games Icons.